Commands in Plugins
Plugin commands allow cTrader users to add custom elements in the following areas:
Add action buttons to the Chart Toolbar. When an action button is clicked, the programmed operation is executed.
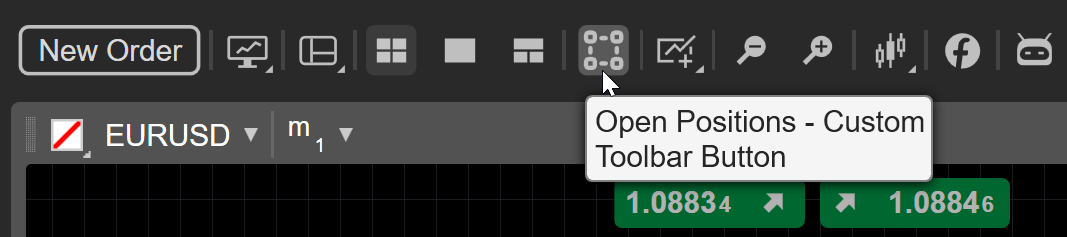
Add buttons with a popup menu to the Chart Toolbar. When such a button is clicked, a menu containing a form, text box or action buttons is displayed.
Sample code:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63 | using System;
using cAlgo.API;
using cAlgo.API.Collections;
using cAlgo.API.Indicators;
using cAlgo.API.Internals;
namespace cAlgo.Plugins
{
[Plugin(AccessRights = AccessRights.None)]
public class CustomToolbarButton : Plugin
{
protected override void OnStart()
{
var icon = new SvgIcon(@"<svg class='w-6 h-6 text-gray-800 dark:text-white' aria-hidden='true' xmlns='http://www.w3.org/2000/svg' fill='none' viewBox='0 0 24 24'>
<path stroke='#BFBFBF' stroke-linecap='round' stroke-linejoin='round' stroke-width='2' d='M11 6.5h2M11 18h2m-7-5v-2m12 2v-2M5 8h2a1 1 0 0 0 1-1V5a1 1 0 0 0-1-1H5a1 1 0 0 0-1 1v2a1 1 0 0 0 1 1Zm0 12h2a1 1 0 0 0 1-1v-2a1 1 0 0 0-1-1H5a1 1 0 0 0-1 1v2a1 1 0 0 0 1 1Zm12 0h2a1 1 0 0 0 1-1v-2a1 1 0 0 0-1-1h-2a1 1 0 0 0-1 1v2a1 1 0 0 0 1 1Zm0-12h2a1 1 0 0 0 1-1V5a1 1 0 0 0-1-1h-2a1 1 0 0 0-1 1v2a1 1 0 0 0 1 1Z'/>
</svg>");
var command = Commands.Add(CommandType.ChartContainerToolbar, OpenPositions, icon);
command.ToolTip = "Open Positions";
Commands.Add(CommandType.ChartContainerToolbar, CloseAllPositions, icon);
}
private CommandResult CloseAllPositions (CommandArgs args)
{
var buttonStyle = new Style();
buttonStyle.Set(ControlProperty.Margin, new Thickness(0, 5, 0, 0));
buttonStyle.Set(ControlProperty.Width, 150);
var closePositionsButton = new Button
{
Text = "Close All Positions",
Style = buttonStyle
};
closePositionsButton.Click += args =>
{
foreach (var position in Positions)
{
position.Close();
}
};
var stackPanel = new StackPanel();
stackPanel.AddChild(closePositionsButton);
return new CommandResult(stackPanel);
}
private void OpenPositions(CommandArgs args)
{
ExecuteMarketOrder(TradeType.Buy, "EURUSD", 1000);
ExecuteMarketOrder(TradeType.Buy, "USDJPY", 1000);
ExecuteMarketOrder(TradeType.Buy, "EURGBP", 1000);
}
protected override void OnStop()
{
// Handle Plugin stop here
}
}
}
|
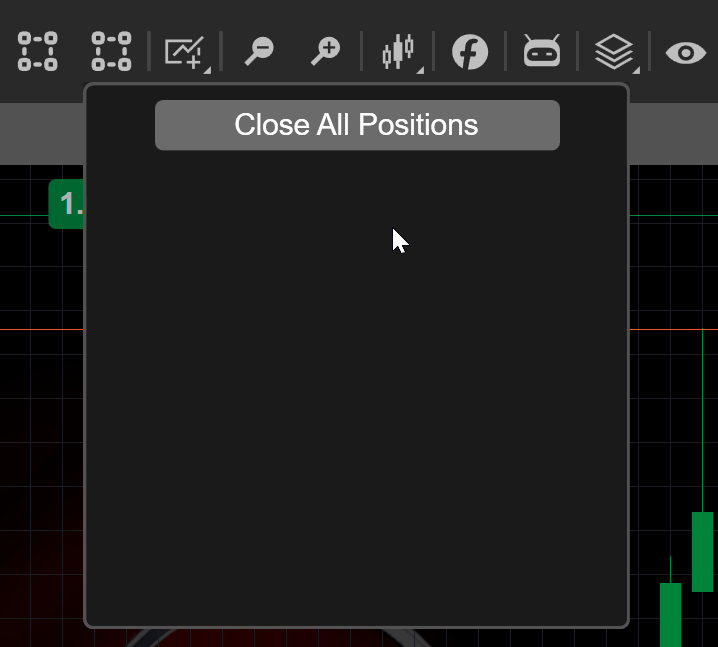
To learn how to create plugins that add action buttons and buttons with popup menus to the Chart Toolbar, see this guide.
Active Symbol Panel (ASP) Commands
Tabs
Add new tabs to display text, numbers, buttons, code, websites or WebView components in the Active Symbol Panel.
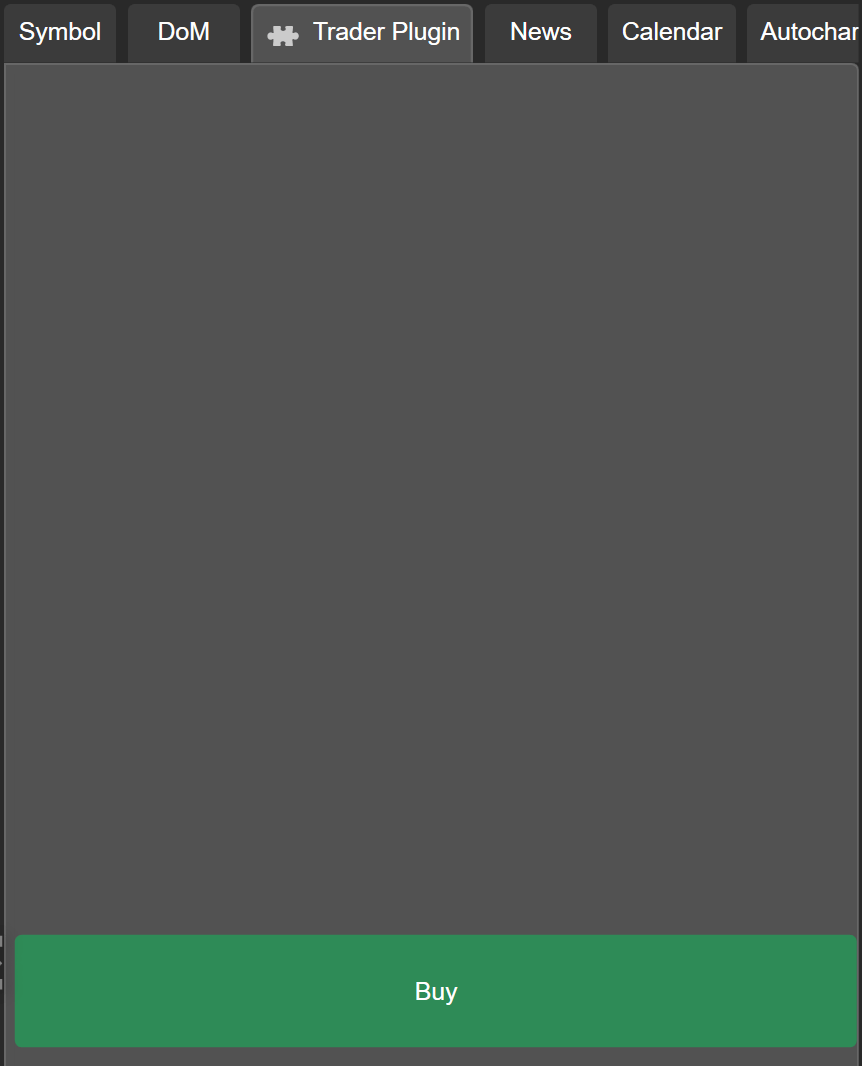
Blocks
Add new blocks to display text, numbers, buttons, code, websites or WebView components in the Active Symbol Panel.
Sample code:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70 | using System;
using System.Linq;
using cAlgo.API;
namespace cAlgo.Plugins
{
[Plugin(AccessRights = AccessRights.None)]
public class MyASPExample : Plugin
{
TextBlock _txtBuyVWAP;
TextBlock _txtSellVWAP;
protected override void OnStart()
{
var block = Asp.SymbolTab.AddBlock("ASP Section Example");
block.Index = 2;
block.Height = 100;
block.IsExpanded = true;
var panel = new StackPanel
{
Orientation = Orientation.Vertical
};
var textBoxStyle = new Style();
textBoxStyle.Set(ControlProperty.Margin, 5);
textBoxStyle.Set(ControlProperty.FontFamily, "Cambria");
textBoxStyle.Set(ControlProperty.FontSize, 16);
textBoxStyle.Set(ControlProperty.Width, 200);
textBoxStyle.Set(ControlProperty.ForegroundColor, Color.Yellow, ControlState.Hover);
_txtBuyVWAP = new TextBlock
{
ForegroundColor = Color.Green,
Text = "Buy Text Box ",
Style = textBoxStyle
};
_txtSellVWAP = new TextBlock
{
ForegroundColor = Color.Red,
Text = "Sell Text Box",
Style = textBoxStyle
};
panel.AddChild(_txtBuyVWAP);
panel.AddChild(_txtSellVWAP);
block.Child = panel;
var buyPositions = Positions.Where(p => p.TradeType == TradeType.Buy);
_txtBuyVWAP.Text = "Buy Positions VWAF: " + Math.Round((buyPositions.Sum(p => p.EntryPrice * p.VolumeInUnits) / buyPositions.Sum(p => p.VolumeInUnits)),5);
var sellPositions = Positions.Where(p => p.TradeType == TradeType.Sell);
_txtSellVWAP.Text = "Sell Positions VWAF: " + Math.Round((sellPositions.Sum(p => p.EntryPrice * p.VolumeInUnits) / sellPositions.Sum(p => p.VolumeInUnits)),5);
Positions.Opened += Positions_Opened;
}
private void Positions_Opened(PositionOpenedEventArgs obj)
{
var buyPositions = Positions.Where(p => p.TradeType == TradeType.Buy);
_txtBuyVWAP.Text = "Buy Positions VWAP: " + (buyPositions.Sum(p => p.EntryPrice * p.VolumeInUnits) / buyPositions.Sum(p => p.VolumeInUnits));
var sellPositions = Positions.Where(p => p.TradeType == TradeType.Sell);
_txtSellVWAP.Text = "Sell Positions VWAP: " + (sellPositions.Sum(p => p.EntryPrice * p.VolumeInUnits) / sellPositions.Sum(p => p.VolumeInUnits));
}
}
}
|
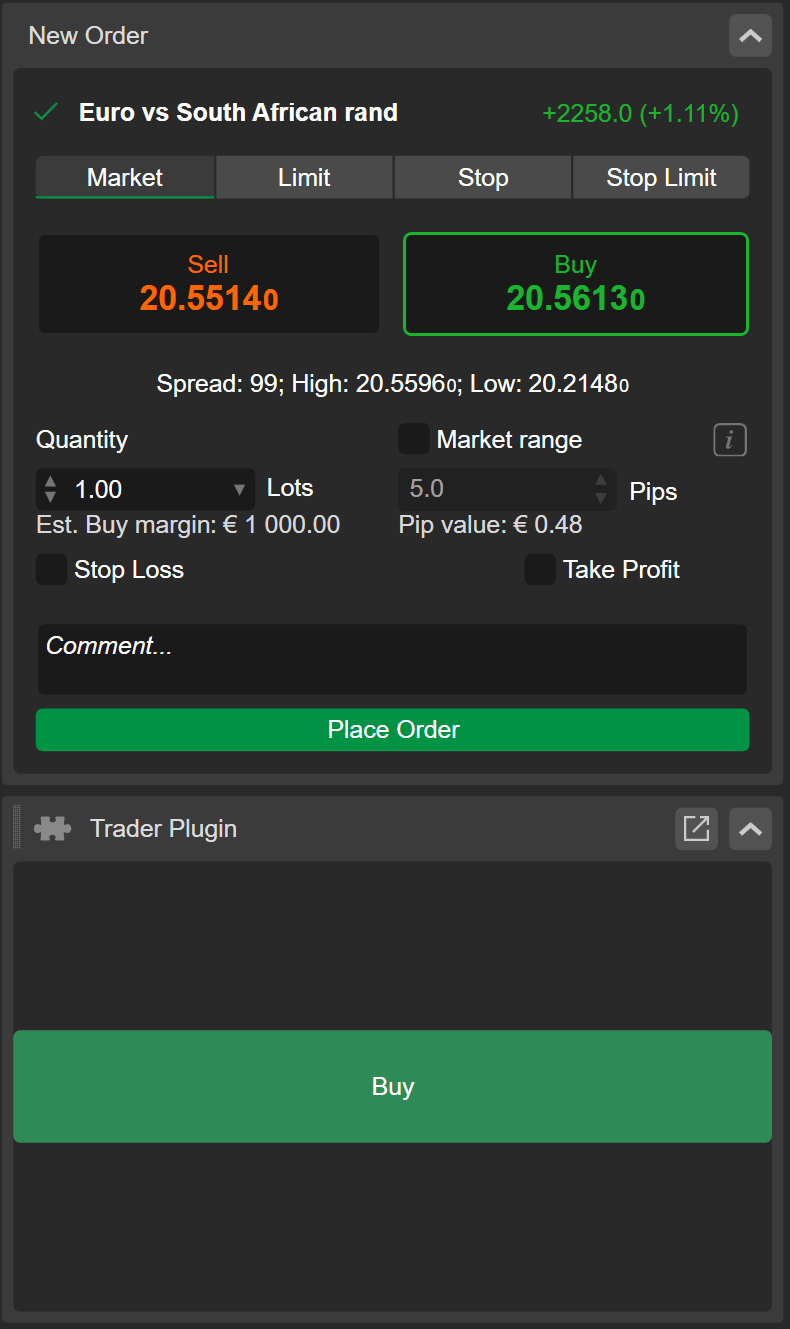
To learn how to create plugins that add websites and VWAP text boxes for open positions, see this guide.
Chart Area Commands
Add custom frames to display text, numbers, websites or WebView components as tabs in the charts area.
Sample code:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34 | using System;
using cAlgo.API;
using cAlgo.API.Collections;
using cAlgo.API.Indicators;
using cAlgo.API.Internals;
namespace cAlgo.Plugins
{
[Plugin(AccessRights = AccessRights.None)]
public class MyCustomFrameExample : Plugin
{
WebView _cTraderWebView = new WebView();
protected override void OnStart()
{
_cTraderWebView.Loaded += _cTraderWebView_Loaded;
var webViewFrame = ChartManager.AddCustomFrame("Forum");
webViewFrame.Child = _cTraderWebView;
webViewFrame.ChartContainer.Mode = ChartMode.Multi;
webViewFrame.Attach();
}
private void _cTraderWebView_Loaded(WebViewLoadedEventArgs args)
{
_cTraderWebView.NavigateAsync("https://ctrader.com/forum");
}
protected override void OnStop()
{
// Handle Plugin stop here
}
}
}
|
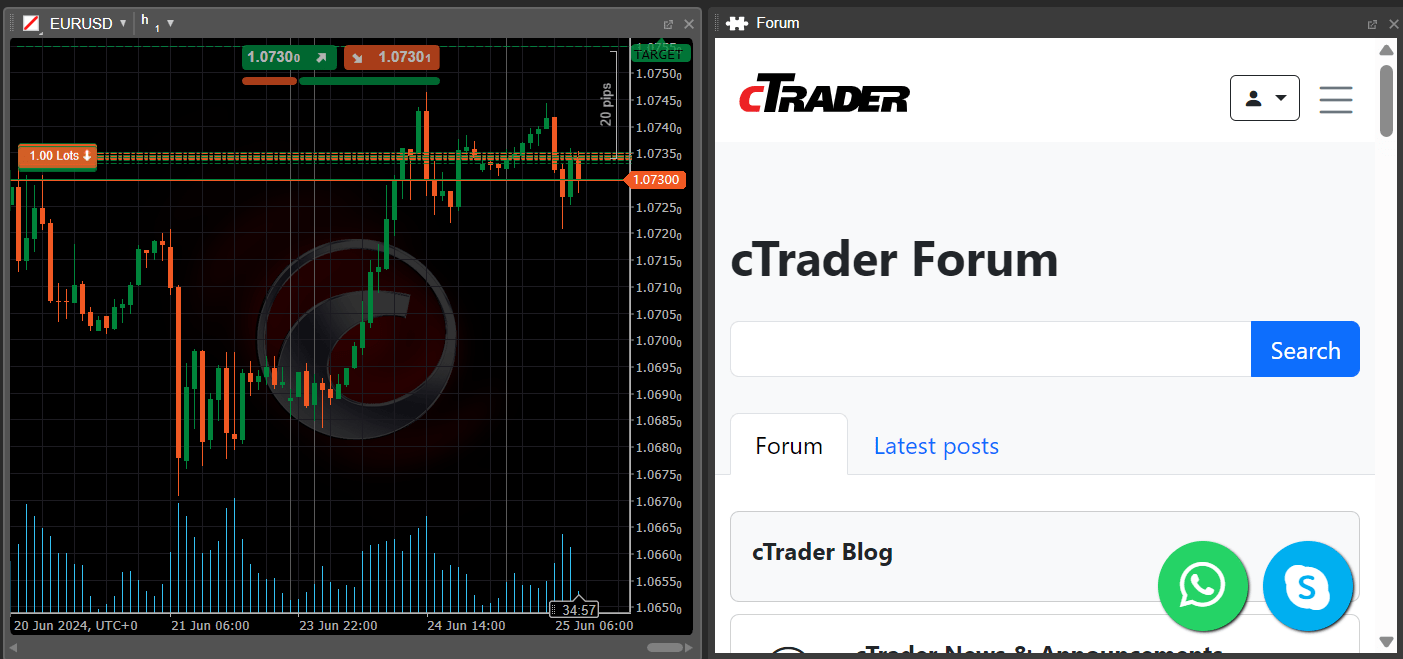
To learn how to create plugins that add websites and text boxes to the chart area, see this guide.
Trade Watch Commands
Add new tabs to display grids, text boxes, websites or WebView components in Trade Watch.
Sample code:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86 | using System;
using cAlgo.API;
using cAlgo.API.Collections;
using cAlgo.API.Indicators;
using cAlgo.API.Internals;
namespace cAlgo.Plugins
{
[Plugin(AccessRights = AccessRights.None)]
public class PreviousBarInfo : Plugin
{
TextBlock _lowBlock;
TextBlock _highBlock;
TextBlock _closeBlock;
TextBlock _openBlock;
Bars _bars;
protected override void OnStart()
{
var tradeWatchTab = TradeWatch.AddTab("Previous Bar Info");
tradeWatchTab.IsSelected = true;
var grid = new Grid(2, 2)
{
HorizontalAlignment = HorizontalAlignment.Center,
VerticalAlignment = VerticalAlignment.Center,
ShowGridLines = true,
Height = 150,
Width = 150,
};
tradeWatchTab.Child = grid;
_bars = MarketData.GetBars(TimeFrame.Minute, "USDJPY");
_lowBlock = new TextBlock
{
Text = "Low:" + _bars.LowPrices.LastValue,
HorizontalAlignment = HorizontalAlignment.Center,
VerticalAlignment = VerticalAlignment.Center,
};
_highBlock = new TextBlock
{
Text = "High:" + _bars.HighPrices.LastValue,
HorizontalAlignment = HorizontalAlignment.Center,
VerticalAlignment = VerticalAlignment.Center,
};
_closeBlock = new TextBlock
{
Text = "Close:" +_bars.ClosePrices.LastValue,
HorizontalAlignment = HorizontalAlignment.Center,
VerticalAlignment = VerticalAlignment.Center,
};
_openBlock = new TextBlock
{
Text = "Open:" + _bars.OpenPrices.LastValue,
HorizontalAlignment = HorizontalAlignment.Center,
VerticalAlignment = VerticalAlignment.Center,
};
grid.AddChild(_lowBlock, 0, 0);
grid.AddChild(_highBlock, 0, 1);
grid.AddChild(_openBlock, 1, 0);
grid.AddChild(_closeBlock, 1, 1);
_bars.Tick += _bars_Tick;
}
private void _bars_Tick(BarsTickEventArgs obj)
{
_lowBlock.Text = "Low: " +_bars.LowPrices.LastValue.ToString();
_highBlock.Text = "High: " +_bars.HighPrices.LastValue.ToString();
_openBlock.Text = "Open: " +_bars.HighPrices.LastValue.ToString();
_closeBlock.Text = "Close: " +_bars.HighPrices.LastValue.ToString();
}
protected override void OnStop()
{
// Handle Plugin stop here
}
}
}
|
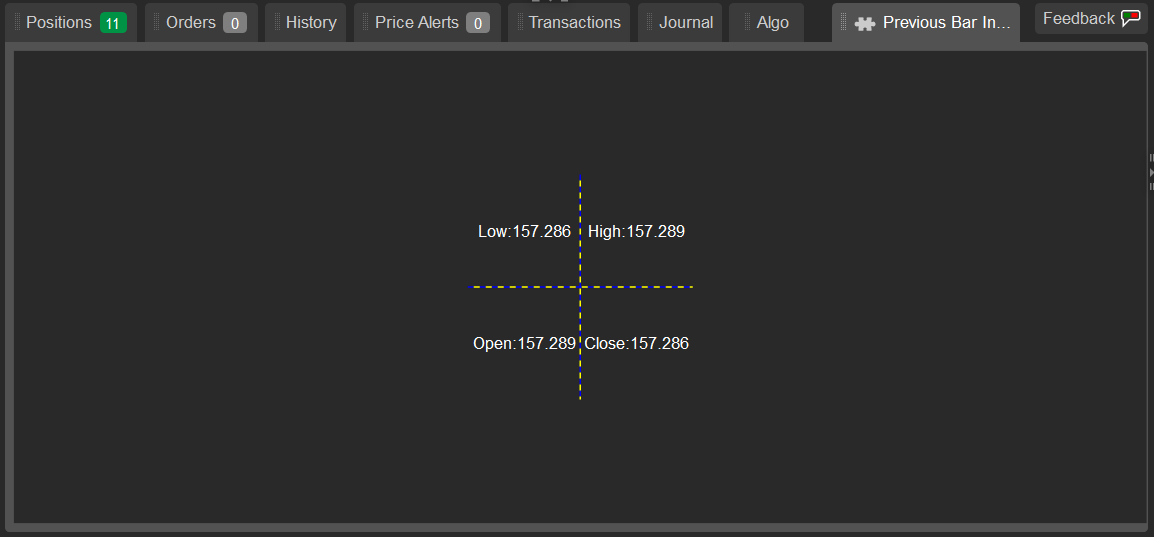
To learn how to create plugins that add websites and informative grids to Trade Watch, see this guide.